代碼實現(xiàn)
Tips:完整源代碼附件:WatchDogDemo-main.zip,就不一步一步寫了
1、創(chuàng)建一個Dog類,主要用于間隔性掃描被看護程序是否還在運行
開了個定時器,每5秒去檢查1次,如果沒有找到進程則使用Process
啟動程序
public class Dog
{
private Timer timer = new Timer();
private string processName ;
private string filePath;//要監(jiān)控的程序的路徑
public Dog()
{
timer.Interval = 5000;
timer.Tick += timer_Tick;
}
public void Start(string filePath)
{
this.filePath = filePath;
this.processName = Path.GetFileNameWithoutExtension(filePath);
timer.Enabled = true;
}
/// <summary>
/// 定時檢測系統(tǒng)是否在運行
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void timer_Tick(object sender, EventArgs e)
{
try
{
Process[] myproc = Process.GetProcessesByName(processName);
if (myproc.Length == 0)
{
Log.Info("檢測到看護程序已退出,開始重新激活程序,程序路徑:{0}",filePath);
ProcessStartInfo info = new ProcessStartInfo
{
WorkingDirectory = Path.GetDirectoryName(filePath),
FileName = filePath,
UseShellexecute = true
};
Process.Start(info);
Log.Info("看護程序已啟動");
}
}
catch (Exception)
{
}
}
}
2、在程序入口接收被看護程序的路徑,啟動Dog
掃描
static class Program
{
static NotifyIcon icon = new NotifyIcon();
private static Dog dog = new Dog();
/// <summary>
/// 應(yīng)用程序的主入口點。
/// </summary>
[STAThread]
static void Main(string[] args)
{
if (args == null || args.Length == 0)
{
MessageBox.Show("啟動參數(shù)異常", "提示", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
string filePath = args[0];
if(!File.Exists(filePath))
{
MessageBox.Show("啟動參數(shù)異常", "提示", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
}
Process current = Process.GetCurrentProcess();
Process[] processes = Process.GetProcessesByName(current.ProcessName);
//遍歷與當(dāng)前進程名稱相同的進程列表
foreach (Process process in processes)
{
//如果實例已經(jīng)存在則忽略當(dāng)前進程
if (process.Id != current.Id)
{
//保證要打開的進程同已經(jīng)存在的進程來自同一文件路徑
if (process.MainModule.FileName.Equals(current.MainModule.FileName))
{
//已經(jīng)存在的進程
return;
}
else
{
process.Kill();
process.WaitForExit(3000);
}
}
}
icon.Text = "看門狗";
icon.Visible = true;
Log.Info("啟動看門狗,看護程序:{0}",filePath);
dog.Start(filePath);
Application.Run();
}
}
3、簡單實現(xiàn)個日志記錄器(使用第三方庫也行,建議看護程序最好不要有任何依賴),也可直接使用我下面這個,很簡單,無任何依賴
public class Log
{
//讀寫鎖,當(dāng)資源處于寫入模式時,其他線程寫入需要等待本次寫入結(jié)束之后才能繼續(xù)寫入
private static ReaderWriterLockSlim LogWriteLock = new ReaderWriterLockSlim();
//日志文件路徑
public static string logPath = "logs\\dog.txt";
//靜態(tài)方法todo:在處理話類型之前自動調(diào)用,去檢查日志文件是否存在
static Log()
{
//創(chuàng)建文件夾
if (!Directory.Exists("logs"))
{
Directory.createDirectory("logs");
}
}
/// <summary>
/// 寫入日志.
/// </summary>
public static void Info(string format, params object[] args)
{
try
{
LogWriteLock.EnterWriteLock();
string msg = args.Length > 0 ? string.Format(format, args) : format;
using (FileStream stream = new FileStream(logPath, FileMode.Append))
{
StreamWriter write = new StreamWriter(stream);
string content = String.Format("{0} {1}",DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss"),msg);
write.WriteLine(content);
//關(guān)閉并銷毀流寫入文件
write.Close();
write.Dispose();
}
}
catch (Exception e)
{
}
finally
{
LogWriteLock.ExitWriteLock();
}
}
}
至此,看護程序已經(jīng)搞定。接著在主程序(被看護程序)封裝一個啟停類
4、主程序封裝看門狗啟停類
public static class WatchDog
{
private static string processName = "WatchDog"; //看護程序進程名(注意這里不是被看護程序名,你可以試一下?lián)Q成主程序名字會使什么效果)
private static string appPath = AppDomain.CurrentDomain.BaseDirectory; //系統(tǒng)啟動目錄
/// <summary>
/// 啟動看門狗
/// </summary>
public static void Start()
{
try
{
string program = string.Format("{0}{1}.exe", appPath, processName);
ProcessStartInfo info = new ProcessStartInfo
{
WorkingDirectory = appPath,
FileName = program,
createNoWindow = true,
UseShellexecute = true,
Arguments = Process.GetCurrentProcess().MainModule.FileName //被看護程序的完整路徑
};
Process.Start(info);
}
catch (Exception)
{
}
}
/// <summary>
/// 停用看門狗
/// </summary>
public static void Stop()
{
Process[] myproc = Process.GetProcessesByName(processName);
foreach (Process pro in myproc)
{
pro.Kill();
pro.WaitForExit(3000);
}
}
}
原理也很簡單,其中有兩點需要注意:
processName
字段表示看護程序,不是被看護程序,如果寫反了,嗯...(你可以試下效果)‘
Arguments
參數(shù)是被看護程序的完整路徑,因為一般情況下,是由被看護程序啟動看護程序,所以我們可以直接使用Process.GetCurrentProcess().MainModule.FileName
獲取到被看護程序的完整路徑
5、在主程序入口點啟動看門狗
public partial class App : Application
{
[STAThread]
static void Main()
{
//程序啟動前調(diào)用看護程序
WatchDog.Start();
Application app = new Application();
MainWindow mainWindow = new MainWindow();
app.Run(mainWindow);
}
}
Winform、普通WPF、Prism等入口點都不太一樣,根據(jù)項目實際情況靈活處理即可
最后在需要正常退出程序的地方(也就是主程序關(guān)閉按鈕或其它想要正常退出程序的地方)停止看門狗程序
效果
https://github.com/luchong0813/WatchDogDemo
后續(xù)
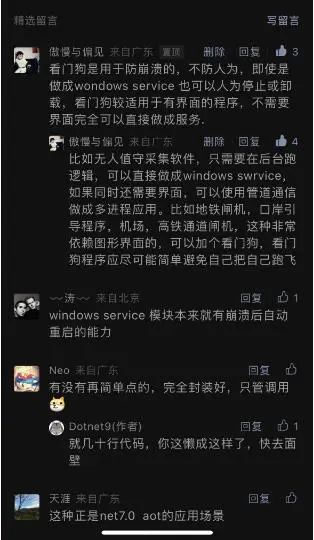
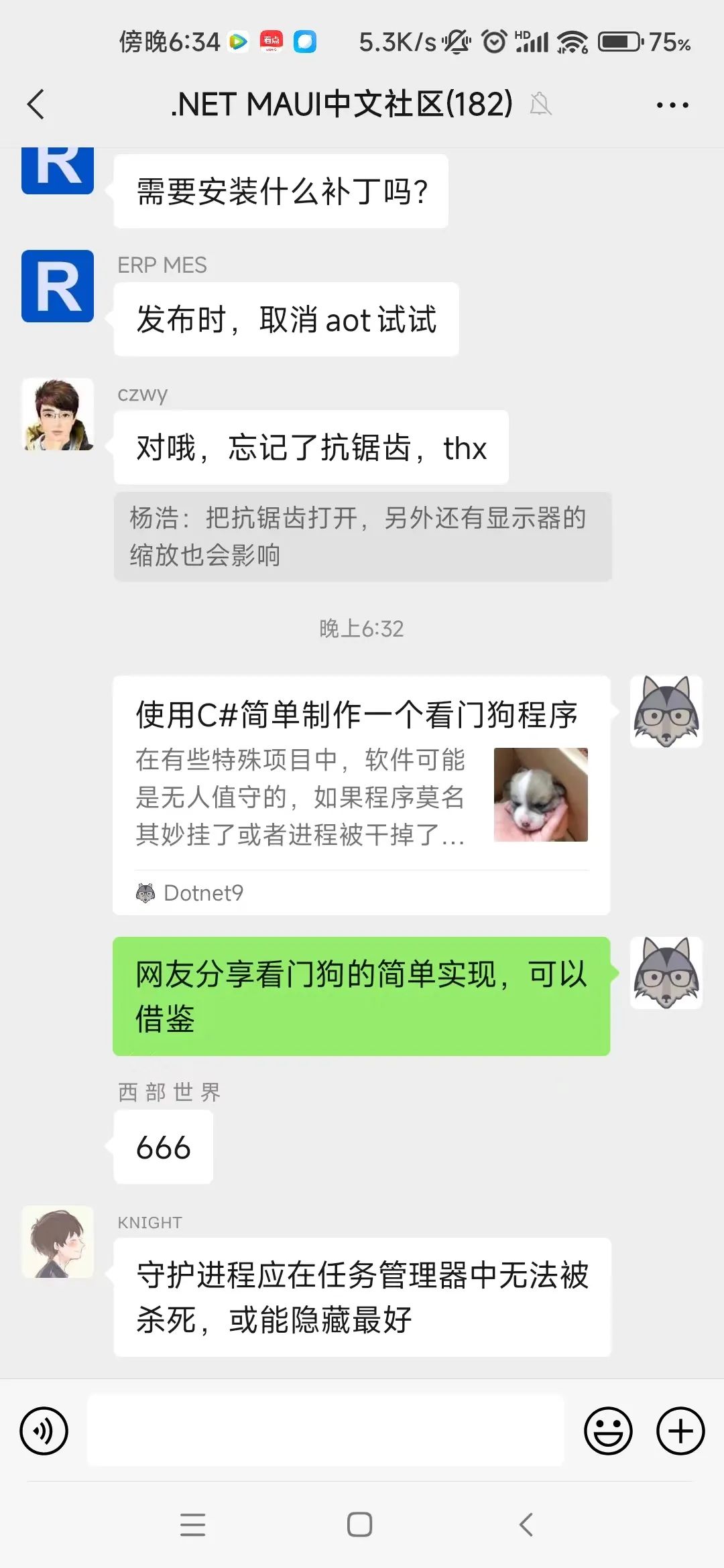
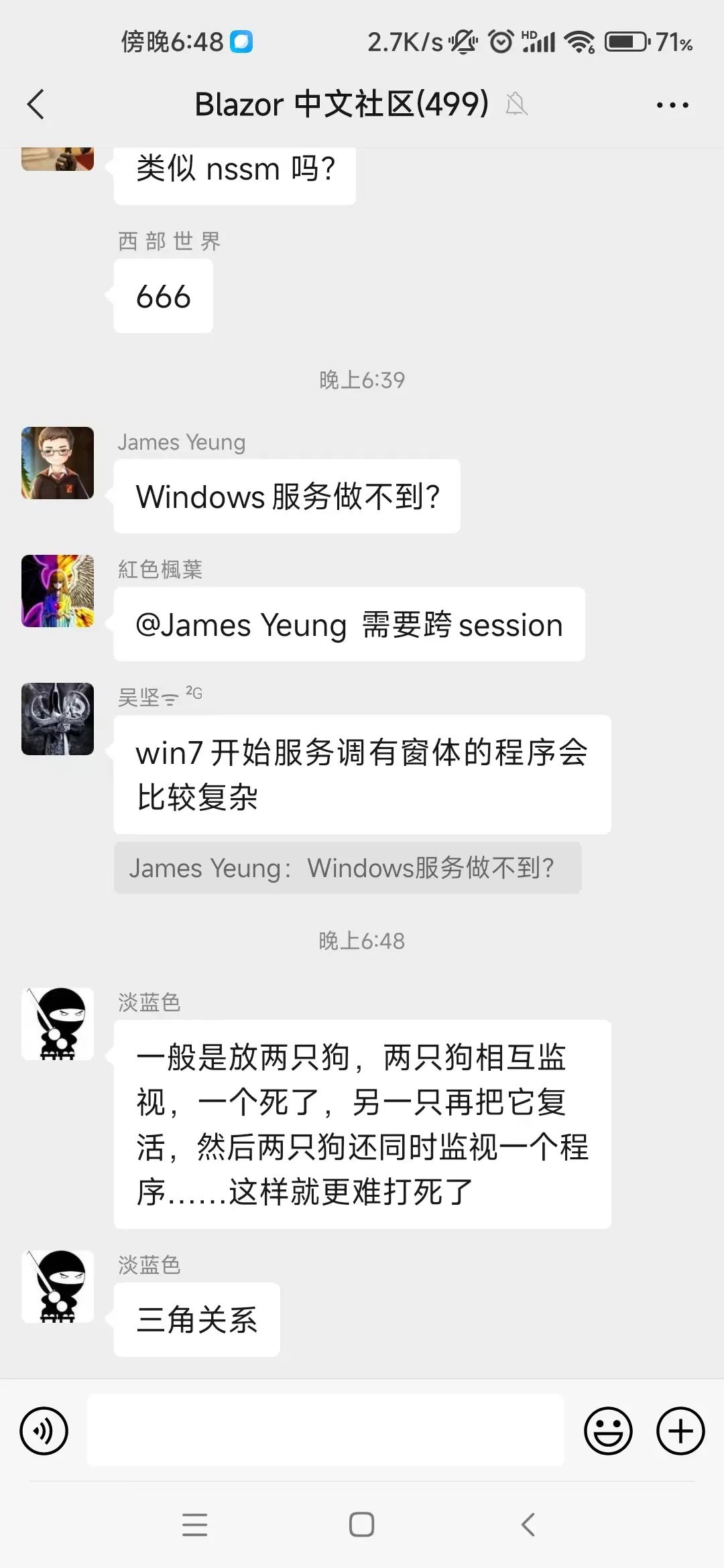
如果是別人的 建議使用nssm
把別人的程序 封裝成服務(wù)由wondows去管理,可以去看我以前發(fā)表的如何用零代碼將應(yīng)用封裝成服務(wù)-NSSM,如果是自己的 需要彈框跟用戶反饋或交互,那就說明不是無人值守,可以不用看門狗。
在類似地鐵進站通道、機場安檢通道、口岸出入境通道等等都有一個引導(dǎo)程序,這種比較依賴圖形界面的可以嘗試使用看門狗程序,看門狗程序不依賴第三方庫且代碼量較少,一般不會跑飛。