LiteDB 是一個輕量、快速、嵌入式的 NoSQL 數(shù)據(jù)庫。它不需要服務(wù)器,適用于桌面、移動應(yīng)用以及小型 Web API 項(xiàng)目,本人在一些小項(xiàng)目中比較喜歡使用。本文將介紹 LiteDB 的主要特點(diǎn)以及如何在 C# 中使用 LiteDB,通過完整的例子展示常見的數(shù)據(jù)庫操作。
LiteDB 主要特點(diǎn)
無需服務(wù)器:LiteDB 運(yùn)行于單一 DLL 中,不需要復(fù)雜的服務(wù)器配置。
輕量級:數(shù)據(jù)庫文件小巧,適用于資源受限的環(huán)境。
嵌入式:LiteDB 直接嵌入到應(yīng)用程序中。
NoSQL:基于 BSON(二進(jìn)制 JSON 序列化協(xié)議)存儲數(shù)據(jù)。
簡單易用:通過簡單的 API 實(shí)現(xiàn) CRUD 操作。
支持 LINQ:方便的數(shù)據(jù)查詢與操作支持。
安裝 LiteDB
首先,通過 NuGet 包管理器安裝 LiteDB??梢栽?Visual Studio 的包管理控制臺中輸入以下命令:
或者在 .csproj
文件中添加以下包引用:
<PackageReference Include="LiteDB" Version="5.0.9" />
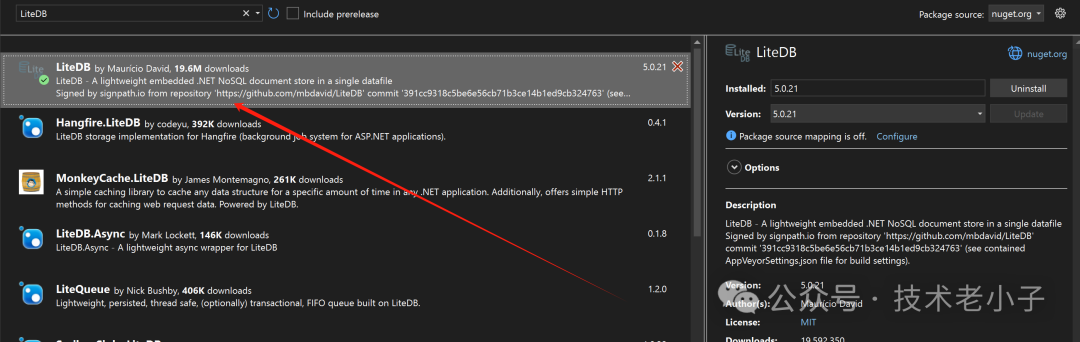
快速開始
我們將通過一個簡單的 C# 控制臺應(yīng)用展示如何使用 LiteDB 實(shí)現(xiàn)基本的 CRUD(創(chuàng)建、讀取、更新、刪除)操作。
1. 創(chuàng)建實(shí)體類
首先,我們需要定義一個實(shí)體類。例如,我們要在數(shù)據(jù)庫中存儲用戶信息,定義如下:
public class User
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
2. 插入數(shù)據(jù)
插入數(shù)據(jù)是基本的數(shù)據(jù)庫操作,我們首先創(chuàng)建 LiteDatabase 實(shí)例,然后獲取集合并插入數(shù)據(jù)。
private void btnInsert_Click(object sender, EventArgs e)
{
// 連接數(shù)據(jù)庫(若文件不存在會自動創(chuàng)建)
using (var db = new LiteDatabase(@"MyData.db"))
{
// 獲取 User 集合(若不存在會自動創(chuàng)建)
var users = db.GetCollection<User>("users");
// 創(chuàng)建新的 User 對象并插入集合
var user = new User { Name = "John Doe", Email = "johndoe@example.com" };
users.Insert(user);
Console.WriteLine("User inserted with Id: " + user.Id);
}
}
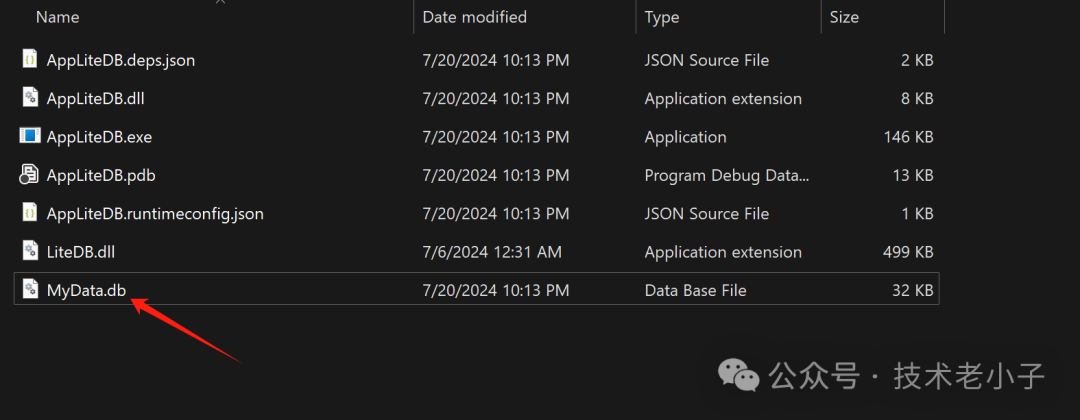
3. 查詢數(shù)據(jù)
LiteDB 提供了簡單的查詢接口,可以使用 LINQ 查詢或通過 BsonExpression 指定查詢條件。
private void btnSearch_Click(object sender, EventArgs e)
{
// 連接數(shù)據(jù)庫
using (var db = new LiteDatabase(@"MyData.db"))
{
var users = db.GetCollection<User>("users");
// 通過 Id 查詢單個用戶
var user = users.FindById(1);
if (user != null)
{
Console.WriteLine($"User found: {user.Name}, {user.Email}");
}
// 使用 LINQ 查詢所有用戶
var allUsers = users.FindAll().ToList();
foreach (var u in allUsers)
{
MessageBox.Show($"User: {u.Name}, {u.Email}");
}
}
}
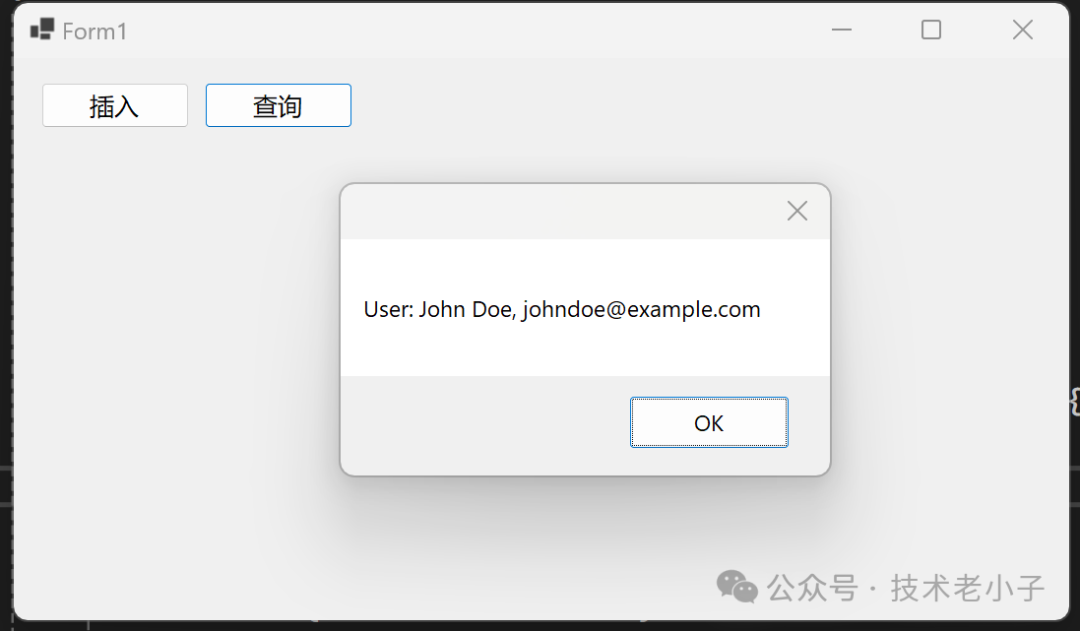
4. 更新數(shù)據(jù)
可以根據(jù) Id 或其他條件查找并更新現(xiàn)有的記錄。
private void btnUpdate_Click(object sender, EventArgs e)
{
// 連接數(shù)據(jù)庫
using (var db = new LiteDatabase(@"MyData.db"))
{
var users = db.GetCollection<User>("users");
// 通過 Id 查找用戶并更新
var user = users.FindById(1);
if (user != null)
{
user.Email = "john.doe@newdomain.com";
users.Update(user);
MessageBox.Show("User updated");
}
}
}
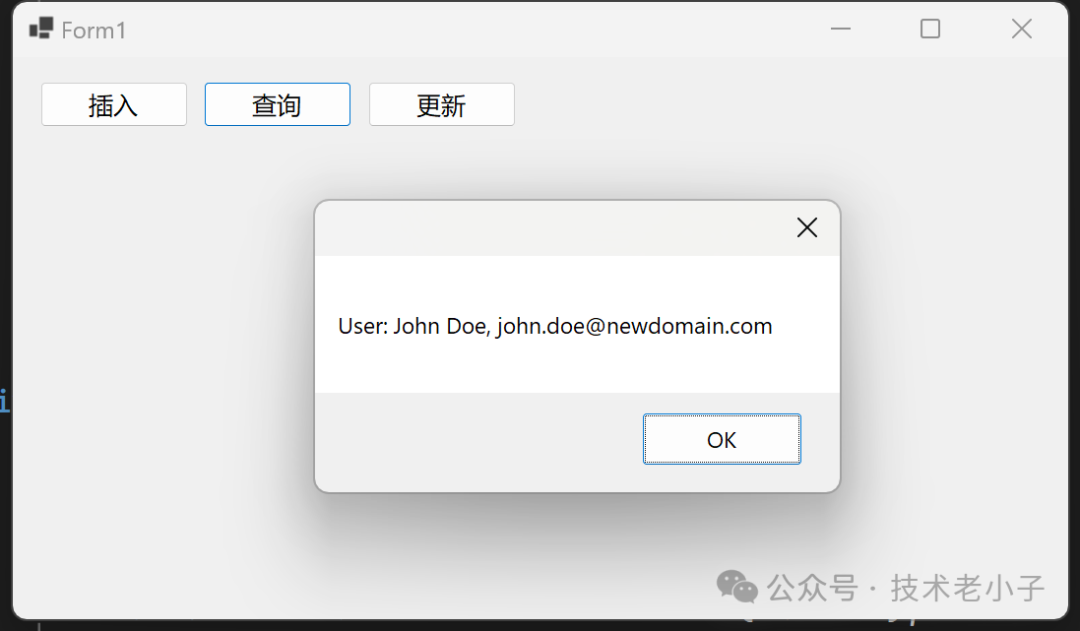
5. 刪除數(shù)據(jù)
可以根據(jù) Id 或其他條件刪除記錄。
private void btnDelete_Click(object sender, EventArgs e)
{
// 連接數(shù)據(jù)庫
using (var db = new LiteDatabase(@"MyData.db"))
{
var users = db.GetCollection<User>("users");
// 通過 Id 刪除用戶
bool success = users.Delete(1);
if (success)
{
MessageBox.Show("User deleted");
}
}
}
定義 IDataRepository 接口
首先,我們定義一個通用的 IDataRepository
接口。這使我們能夠以更抽象的方式處理數(shù)據(jù)存儲庫,并方便以后進(jìn)行擴(kuò)展。
public interface IDataRepository<T>
{
void Insert(T entity);
T GetById(int id);
IEnumerable<T> GetAll();
void Update(T entity);
bool Delete(int id);
}
實(shí)現(xiàn) UserRepository 類
接下來,我們實(shí)現(xiàn)一個 UserRepository
類來處理 User
實(shí)體的數(shù)據(jù)庫操作。UserRepository
類繼承 IDataRepository<User>
接口。
using LiteDB;
using System;
using System.Collections.Generic;
public class UserRepository : IDataRepository<User>, IDisposable
{
private readonly LiteDatabase _database;
private readonly ILiteCollection<User> _usersCollection;
public UserRepository(string databasePath)
{
_database = new LiteDatabase(databasePath);
_usersCollection = _database.GetCollection<User>("users");
}
public void Insert(User entity)
{
_usersCollection.Insert(entity);
}
public User GetById(int id)
{
return _usersCollection.FindById(id);
}
public IEnumerable<User> GetAll()
{
return _usersCollection.FindAll();
}
public void Update(User entity)
{
_usersCollection.Update(entity);
}
public bool Delete(int id)
{
return _usersCollection.Delete(id);
}
public void Dispose()
{
_database?.Dispose();
}
}
使用 UserRepository 類
有了數(shù)據(jù)倉儲類之后,我們可以在應(yīng)用程序中使用 UserRepository
來進(jìn)行User
實(shí)體的數(shù)據(jù)庫操作。
private void btnRepository_Click(object sender, EventArgs e)
{
// 數(shù)據(jù)庫文件路徑
string databasePath = @"MyData.db";
using (var userRepository = new UserRepository(databasePath))
{
// 插入數(shù)據(jù)
var newUser = new User { Name = "Jane Doe", Email = "janedoe@example.com" };
userRepository.Insert(newUser);
MessageBox.Show($"User inserted with Id: {newUser.Id}");
// 查詢數(shù)據(jù)
var user = userRepository.GetById(newUser.Id);
if (user != null)
{
MessageBox.Show($"User found: {user.Name}, {user.Email}");
}
// 更新數(shù)據(jù)
user.Email = "jane.doe@newdomain.com";
userRepository.Update(user);
MessageBox.Show("User updated");
// 列出所有用戶
var allUsers = userRepository.GetAll();
foreach (var u in allUsers)
{
MessageBox.Show($"User: {u.Name}, {u.Email}");
}
// 刪除數(shù)據(jù)
if (userRepository.Delete(user.Id))
{
MessageBox.Show("User deleted");
}
}
}
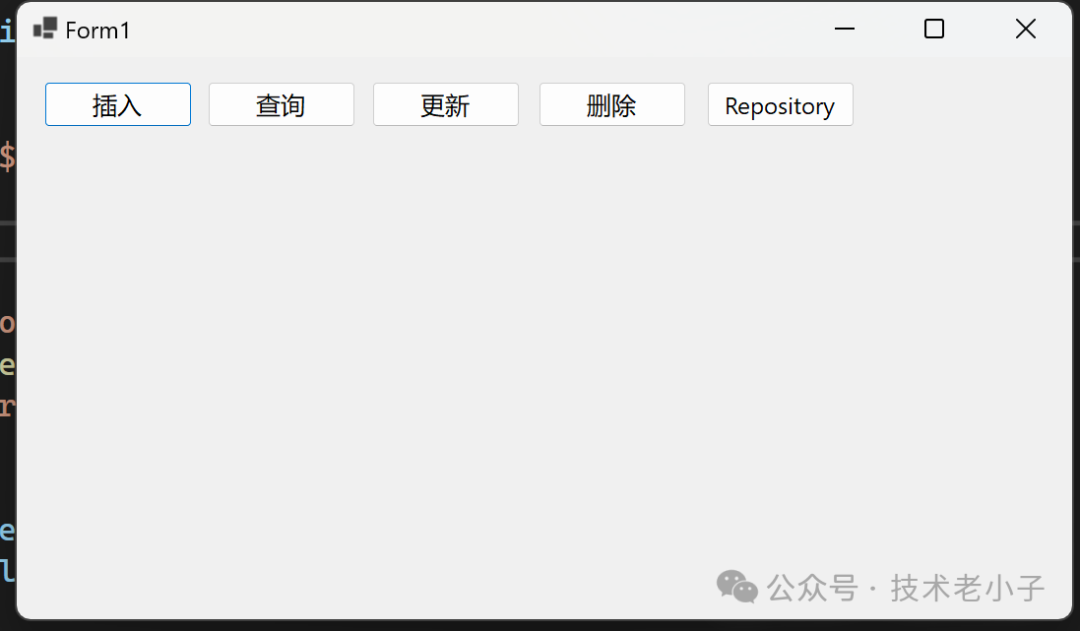
結(jié)論
LiteDB 是一個非常實(shí)用且易于使用的嵌入式 NoSQL 數(shù)據(jù)庫,適用于小型項(xiàng)目和快速開發(fā)。在 C# 中使用 LiteDB 非常簡單且高效,通過簡單的 API,我們可以方便地進(jìn)行數(shù)據(jù)存儲和查詢。本文介紹了 LiteDB 的基本特點(diǎn),并通過完整的例子展示了如何進(jìn)行 CRUD 操作,幫助開發(fā)者快速上手。
該文章在 2024/8/19 18:43:32 編輯過