?
英文 | https://medium.com/@terawuthTh/7-most-commonly-used-vue-3-f7a70b9080c7
介紹:
由于我在工作的公司中角色和職責(zé)的變化,作為后端開發(fā)人員的我在去年年底選擇了 Vue.js。當(dāng)我深入研究時,我發(fā)現(xiàn) Vue.js 非常有趣。它不像 Angular 那樣有很高的學(xué)習(xí)曲線,而且比 React 更輕量和靈活。
Vue.js 絕對是一個用于構(gòu)建用戶界面的強大 JavaScript 框架。為了創(chuàng)建具有視覺吸引力的交互式用戶界面,合并 UI 組件非常重要。
在這篇文章中,我將介紹我在工作中經(jīng)常使用的 7 個 UI 組件。我還將介紹它們的目的、實施示例和現(xiàn)實生活中的用例。
UI 組件的重要性
UI 組件在 Vue.js 開發(fā)中發(fā)揮著重要作用。通過將復(fù)雜的接口分解為模塊化、可重用的組件,開發(fā)人員可以創(chuàng)建可擴展且高效的代碼,更易于維護和故障排除。
此外,合并 UI 組件還可以改善用戶體驗和界面一致性,因為用戶將在不同的頁面或應(yīng)用程序中獲得熟悉的體驗。
Vue.js 7 個最常用的 UI 組件
1. 按鈕組件
按鈕使用戶能夠與應(yīng)用程序交互。在網(wǎng)站上看不到按鈕幾乎是不可能的。它可能是最簡單的組件之一,但其用途卻并非如此。這是網(wǎng)站的號召性用語。因此,請仔細思考如何使其脫穎而出,如何處理不同的狀態(tài)以及如何使用它來驗證某些操作。
現(xiàn)實項目中的示例:注冊表單的提交按鈕、電子商務(wù)網(wǎng)站上的“添加到購物車”按鈕。
這是一個簡單的例子: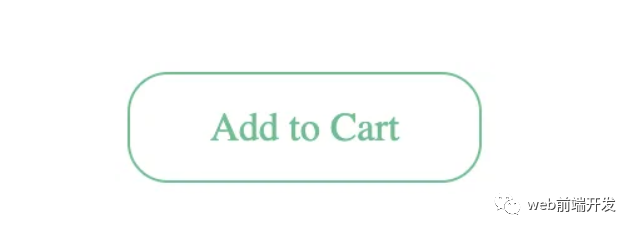
<template>
<button>{{props.title || 'Add To Cart'}}</button>
</template>
<script setup>
const props = defineProps(['title']);
</script>
<style>
button {
color: #4fc08d;
}
button {
background: none;
border: solid 1px;
border-radius: 1em;
font: inherit;
padding: 0.65em 2em;
}
</style>
演示:https://codepen.io/terawuth/pen/poqRJvZ
2. 表單組件
表單用于收集 Web 應(yīng)用程序中的用戶輸入,并且可以針對不同的輸入類型(文本、電子郵件、密碼等)進行自定義。 在網(wǎng)站上很難不看到表單。 驗證、步驟或指南等元素對于改善表單填寫體驗大有幫助。
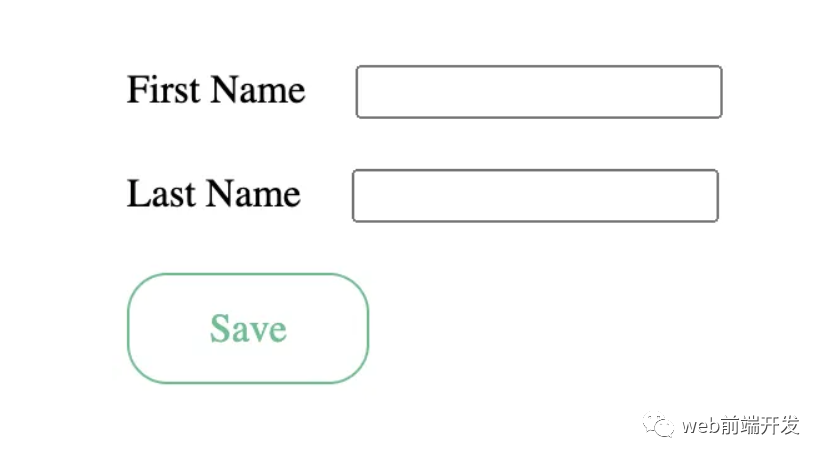
現(xiàn)實項目中的示例:用于注冊、聯(lián)系或登錄的用戶輸入表單。
<template>
<div>
<form>
<div>
<label>First Name</label>
<input type="text" name="first_name" v-model="firstName" />
</div>
<div>
<label>Last Name</label>
<input type="text" name="last_name" v-model="lastName" />
</div>
<div>
<Button @click="onSave" title="Save" />
</div>
</form>
</div>
</template>
<script setup>
import { ref } from "vue";
import Button from "https://codepen.io/terawuthth/pen/poqRJvZ.js";
const firstName = ref("");
const lastName = ref("");
const onSave = () => alert("Save !!");
</script>
<style>
form {
display: flex;
flex-direction: column;
}
.form-item input {
margin-left: 20px
}
.form-item {
margin-top: 20px;
}
</style>
演示:https://codepen.io/terawuth/pen/KKbzLzK
3.卡片組件
卡片用于以視覺上吸引人的方式組織和顯示信息。如今,卡片組件非常常見,尤其是在社交媒體網(wǎng)站上。盡管卡片組件以一種美觀且干凈的方式呈現(xiàn)信息,但必須小心不要在卡片上放置太多數(shù)據(jù)。
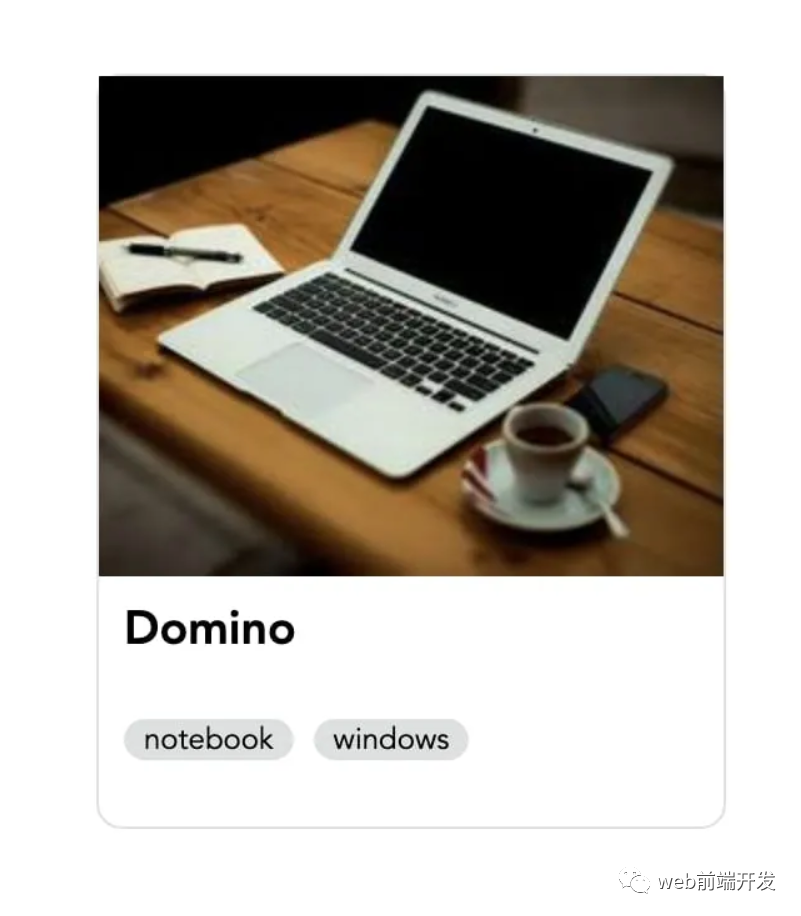
現(xiàn)實項目中的示例:網(wǎng)站上的新聞文章卡、社交媒體資料卡。
<template>
<div>
<div>
<img :src="product.image" alt="Product" />
</div>
<div>
<div>
<h3>
<a :href="product.link">{{ product.name }}</a>
</h3>
</div>
<div>
<a v-for="tag in tags" :href="tag.link">
<span>{{ tag.name }}</span>
</a>
</div>
</div>
</div>
</template>
<script setup>
import { ref, reactive } from "vue";
const tags = ref([
{
link: "#",
name: "notebook"
},
{
link: "#",
name: "windows"
}
]);
const product = reactive({
name: "Domino",
image:
"https://picsum.photos/id/2/250/200",
link: "#"
});
</script>
<style>
.card {
width: 250px;
height: 300px;
border: 1px solid #dedfdf;
border-radius: 10px;
}
.card:hover {
border: 2px solid #adaeae;
box-shadow: 2px 2px #adaeae;
}
.card .img-product {
height: 60%;
margin: 0;
}
.card .img-product img {
height: 200px;
width: 100%;
}
.detail {
height: 40%;
padding: 10px;
display: flex;
flex-direction: column;
justify-content: start;
align-items: start;
}
.tag a {
color: black;
background-color: #dedfdf;
padding: 0 8px 0 8px;
border-radius: 20px;
text-decoration: none;
}
.tag a:not(:first-child) {
margin-left: 8px;
}
.detail a {
color: black;
text-decoration: none;
}
.detail .tag a:hover {
color: #fff;
background-color: #fc6969;
}
.detail .title h3:hover {
color: #fc6969;
}
</style>
演示:https://codepen.io/terawuth/pen/XWogWqW
4. 導(dǎo)航組件
導(dǎo)航允許用戶導(dǎo)航到應(yīng)用程序內(nèi)的不同部分或頁面。大多數(shù)網(wǎng)站至少有 1 個菜單。菜單對于引導(dǎo)用戶非常重要,但太多菜單或不正確的菜單分組可能會導(dǎo)致混亂。

現(xiàn)實項目中的示例:公司網(wǎng)站的導(dǎo)航欄、餐廳網(wǎng)站的菜單。
<template>
<div>
<a
v-for="menu in menuList"
:class="{ active: menu.name === activeMenu }"
:href="menu.link"
>
{{ menu.name }}
</a>
</div>
</template>
<script setup>
import { ref } from "vue";
const activeMenu = ref("menu1");
const menuList = ref([
{
link: "#",
name: "menu1"
},
{
link: "#",
name: "menu2"
},
{
link: "#",
name: "menu3"
}
]);
</script>
<style>
body {
margin: 0;
padding: 0;
}
.nav {
overflow: hidden;
background-color: #333;
}
.nav a {
float: left;
color: #f2f2f2;
text-align: center;
padding: 14px 16px;
text-decoration: none;
font-size: 17px;
}
.nav a:hover {
background-color: #ddd;
color: black;
}
.nav a.active {
background-color: #04aa6d;
color: white;
}
</style>
演示:https://codepen.io/terawuth/pen/bGOwJvo
5. 模態(tài)組件
模態(tài)框用于在主要內(nèi)容之上的彈出窗口中顯示內(nèi)容。它非常適合在不進入新頁面的情況下顯示有意義的信息。雖然它可以讓用戶專注于特定的地方,但它可能會讓人厭煩。一個例子是廣告模式。
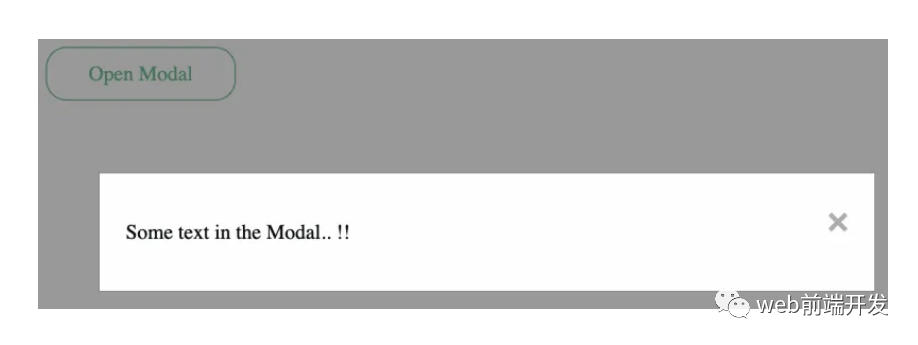
現(xiàn)實項目中的示例:登錄或注冊彈出窗口、確認消息。
<template>
<div>
<Button @click="showModal" title="Open Modal" />
<!-- The Modal -->
<div id="myModal" class="modal" :style="{ display: modalDisplay }">
<div class="modal-content">
<span class="close" @click="closeModal">×</span>
<p>{{ content }}</p>
</div>
</div>
</div>
</template>
<script setup>
import { ref } from "vue";
import Button from "https://codepen.io/terawuthth/pen/poqRJvZ.js";
const modalDisplay = ref("none");
const content = ref("Some text in the Modal.. !!");
const showModal = () => {
modalDisplay.value = "block";
};
const closeModal = () => (modalDisplay.value = "none");
</script>
<style>
.modal {
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgb(0, 0, 0);
background-color: rgba(0, 0, 0, 0.4);
}
.modal-content {
background-color: #fefefe;
margin: 15% auto;
padding: 20px;
border: 1px solid #888;
width: 80%;
}
.close {
color: #aaa;
float: right;
font-size: 28px;
font-weight: bold;
}
.close:hover,
.close:focus {
color: black;
text-decoration: none;
cursor: pointer;
}
</style>
演示:https://codepen.io/terawuth/pen/gOZRRdo
6. 警報組件
警報提供反饋并通知用戶有關(guān)應(yīng)用程序中的重要信息或事件。警報是一種非常有用的方式來反饋用戶而不打擾他們。但我們應(yīng)該考慮何時使用一段時間后消失的警報或何時需要用戶關(guān)閉警報。
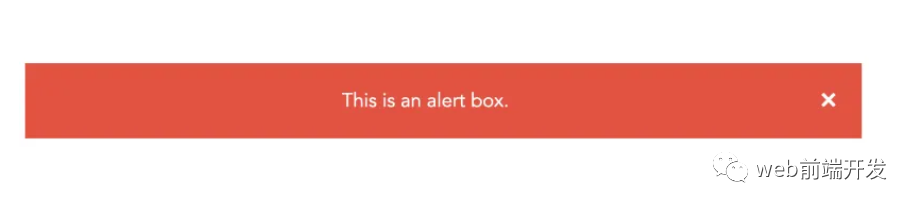
現(xiàn)實項目中的示例:付款或交易的成功或錯誤消息、站點維護通知。
<template>
<div class="alert" :style="{ display: alertDisplay }">
<span
class="closebtn"
@click="closeAlert"
onclick="this.parentElement.style.display='none';"
>
×
</span>
This is an alert box.
</div>
</template>
<script setup>
import { ref } from "vue";
const isAlert = ref(true);
const alertDisplay = ref("block");
const closeAlert = () => (alertDisplay.value = "none");
</script>
<style>
.alert {
padding: 20px;
background-color: #f44336;
color: white;
margin-bottom: 15px;
}
.closebtn {
margin-left: 15px;
color: white;
font-weight: bold;
float: right;
font-size: 22px;
line-height: 20px;
cursor: pointer;
transition: 0.3s;
}
.closebtn:hover {
color: black;
}
</style>
演示:https://codepen.io/terawuth/pen/WNLOyOo
7.頭像組件
頭像是代表應(yīng)用程序中用戶的圖標(biāo)或圖像。它們使用戶帳戶更加個性化并且易于用戶識別。
但是,并非所有系統(tǒng)都需要用戶帳戶具有圖標(biāo)或圖像。如果用戶不上傳它們,我們應(yīng)該提供默認圖像或使用他們名字的首字母以提供更好的用戶體驗。
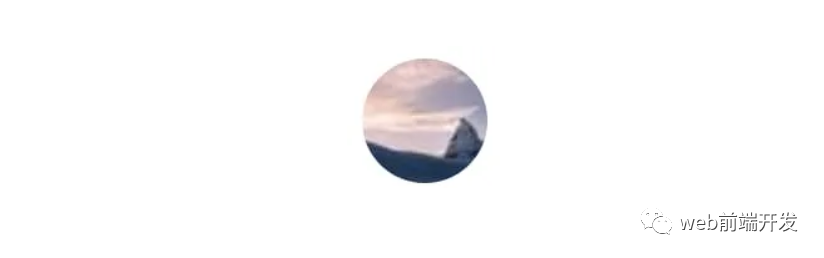
現(xiàn)實項目中的示例:社交媒體網(wǎng)站或聊天應(yīng)用程序上的用戶個人資料。
<template>
<div class="avatar">
<img :src="imgPath" id="img-avatar" alt="Avatar" />
</div>
</template>
<script setup>
import { ref } from "vue";
const imgPath = ref(
"https://picsum.photos/id/866/50/50"
);
</script>
<style scoped>
.avatar {
vertical-align: middle;
width: 50px;
height: 50px;
}
#img-avatar {
border-radius: 50%;
}
</style>
演示:https://codepen.io/terawuth/pen/PoXJeqK
注意:您可以在此地址:https://codepen.io/collection/bNmVjq中找到 7 個 UI 組件集合的代碼。
優(yōu)點和注意事項
在 Vue.js 開發(fā)中使用這些 UI 組件的好處包括:
然而,考慮一些限制或缺點也很重要,例如,由于頁面上有大量組件而導(dǎo)致的潛在性能問題,或者需要超出預(yù)構(gòu)建組件提供范圍的自定義。 因此,在使用預(yù)構(gòu)建組件和定制代碼之間取得平衡非常重要。就我而言,我來自使用 Node.js(基于 Javascript)的后端開發(fā)。 我不必同時學(xué)習(xí)新語言和新框架。
此外,Vue.js 文檔鼓勵新手使用函數(shù)式編程原則(例如 Composition API)進行開發(fā)。 同樣,鼓勵后端開發(fā)人員盡可能使用這個概念,從而使向前端開發(fā)的過渡變得更容易。
結(jié)論
Vue.js 是一個用于開發(fā)引人入勝的用戶界面的強大工具,合并 UI 組件可以將您的項目提升到一個新的水平。 這些只是我個人對 7 個最常用 UI 組件的看法,開發(fā)人員可以創(chuàng)建用戶喜歡的可擴展、高效且具有視覺吸引力的界面。
作為一個剛接觸 Vue.js 的后端開發(fā)人員,我還有很多東西需要學(xué)習(xí)。 希望通過分享我的經(jīng)驗旅程,可以鼓勵任何有興趣學(xué)習(xí)和擴展對 Vue.js 中 UI 組件開發(fā)的理解的人。
該文章在 2024/10/14 10:09:12 編輯過