FluentFTP 是一個功能豐富的 .NET FTP 客戶端庫,它提供了一個簡單而直觀的 API 來執(zhí)行各種 FTP 操作。本文將詳細介紹 FluentFTP 的使用方法,并提供多個實用的例子。
1. 安裝
首先,通過 NuGet 包管理器安裝 FluentFTP:
Install-Package FluentFTP
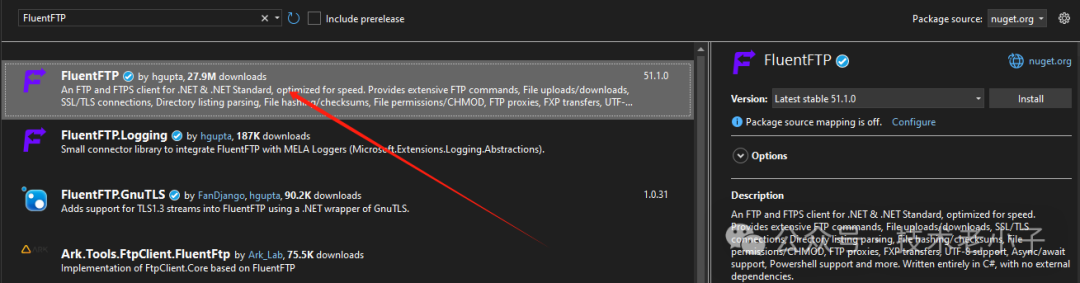
2. 基本用法
2.1 連接到 FTP 服務器
static async Task Main(string[] args)
{
? ?using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
? ?{
? ? ? ?await client.Connect();
? ? ? ?Console.WriteLine("Connected to FTP server!");
? ? ? ?// 執(zhí)行其他操作...
? ? ? ?await client.Disconnect();
? ?}
}

2.2 列出目錄內容
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?foreach (var item in await client.GetListing("/"))
? ?{
? ? ? ?Console.WriteLine($"{item.Name} - {item.Modified} - {item.Size} bytes");
? ?}
}
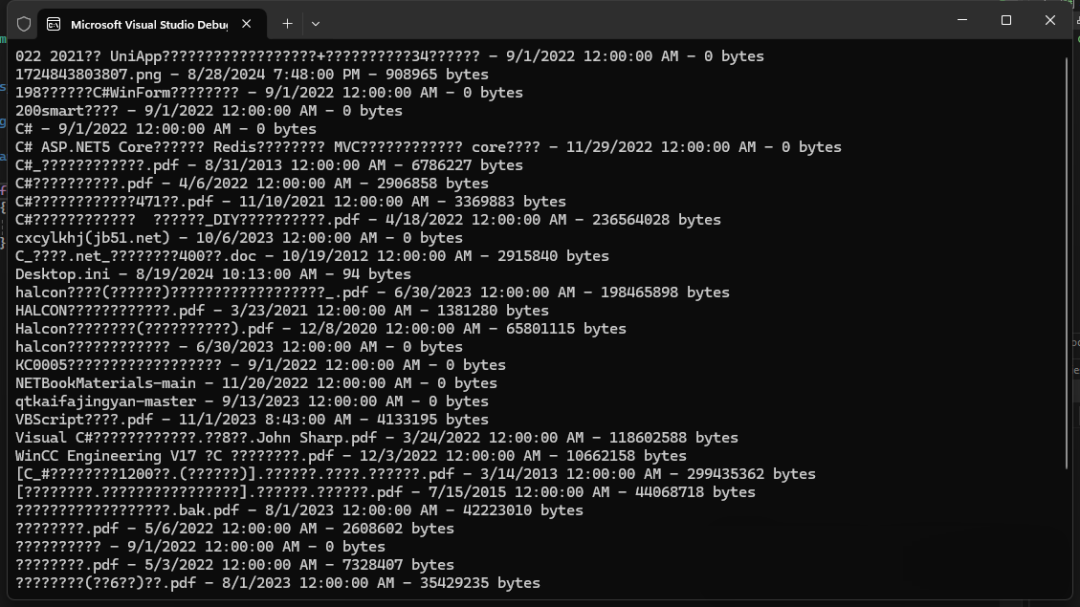
中文亂碼
static async Task Main(string[] args)
{
? ?// 注冊編碼提供程序 ?
? ?Encoding.RegisterProvider(CodePagesEncodingProvider.Instance);
? ?// 確??刂婆_可以顯示正確的字符 ?
? ?Console.OutputEncoding = Encoding.GetEncoding("GB2312");
? ?using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
? ?{
? ? ? ?// 設置FTP客戶端使用GB2312編碼 ?
? ? ? ?client.Encoding = Encoding.GetEncoding("GB2312");
? ? ? ?await client.Connect();
? ? ? ?foreach (var item in await client.GetListing("/"))
? ? ? ?{
? ? ? ? ? ?Console.WriteLine($"{item.Name} - {item.Modified} - {item.Size} bytes");
? ? ? ?}
? ?}
}
?
2.3 上傳文件
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var status = await client.UploadFile(@"C:\local\file.txt", "/remote/file.txt");
? ?if (status == FtpStatus.Success)
? ?{
? ? ? ?Console.WriteLine("File uploaded successfully!");
? ?}
}
2.4 下載文件
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var status = await client.DownloadFile(@"D:\output\1724843803807.png", "1724843803807.png");
? ?if (status == FtpStatus.Success)
? ?{
? ? ? ?Console.WriteLine("File downloaded successfully!");
? ?}
}
3. 高級用法
3.1 創(chuàng)建目錄
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var created = await client.CreateDirectory("/new_directory");
? ?if (created)
? ?{
? ? ? ?Console.WriteLine("Directory created successfully!");
? ?}
}
3.2 刪除文件
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var deleted = await client.DeleteFile("/remote/file_to_delete.txt");
? ?if (deleted)
? ?{
? ? ? ?Console.WriteLine("File deleted successfully!");
? ?}
}
3.3 重命名文件或目錄
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var renamed = await client.Rename("/old_name.txt", "/new_name.txt");
? ?if (renamed)
? ?{
? ? ? ?Console.WriteLine("File renamed successfully!");
? ?}
}
3.4 檢查文件是否存在
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var exists = await client.FileExists("/remote/file.txt");
? ?Console.WriteLine($"File exists: {exists}");
}
3.5 獲取文件大小
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var size = await client.GetFileSize("/remote/file.txt");
? ?Console.WriteLine($"File size: {size} bytes");
}
3.6 上傳整個目錄
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var result = await client.UploadDirectory(@"C:\local\directory", "/remote/directory");
? ?Console.WriteLine($"Uploaded {result.UploadedFiles.Count} files");
? ?Console.WriteLine($"Failed to upload {result.FailedUploads.Count} files");
}
3.7 下載整個目錄
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?await client.Connect();
? ?var result = await client.DownloadDirectory(@"C:\local\directory", "/remote/directory");
? ?Console.WriteLine($"Downloaded {result.DownloadedFiles.Count} files");
? ?Console.WriteLine($"Failed to download {result.FailedDownloads.Count} files");
}
3.8 使用 FTPS(FTP over SSL)
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?client.Config.EncryptionMode = FtpEncryptionMode.Explicit;
? ?client.Config.ValidateAnyCertificate = true; // 在生產環(huán)境中應該使用proper證書驗證
? ?await client.Connect();
? ?Console.WriteLine("Connected to FTPS server!");
? ?// 執(zhí)行其他操作...
}
3.9 設置傳輸模式
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?client.Config.DataConnectionType = FtpDataConnectionType.PASV; // 使用被動模式
? ?await client.Connect();
? ?// 執(zhí)行其他操作...
}
4. 錯誤處理
FluentFTP 使用異常來處理錯誤。以下是一個錯誤處理的例子:
using (var client = new AsyncFtpClient("127.0.0.1", "admin", "123456"))
{
? ?try
? ?{
? ? ? ?await client.Connect();
? ? ? ?await client.UploadFile(@"C:\local\file.txt", "/remote/file.txt");
? ? ? ?Console.WriteLine("File uploaded successfully!");
? ?}
? ?catch (FtpAuthenticationException ex)
? ?{
? ? ? ?Console.WriteLine($"Authentication failed: {ex.Message}");
? ?}
? ?catch (FtpException ex)
? ?{
? ? ? ?Console.WriteLine($"FTP error occurred: {ex.Message}");
? ?}
? ?catch (Exception ex)
? ?{
? ? ? ?Console.WriteLine($"An error occurred: {ex.Message}");
? ?}
? ?finally
? ?{
? ? ? ?await client.Disconnect();
? ?}
}
5. 結論
FluentFTP 是一個功能豐富且易于使用的 FTP 客戶端庫。它提供了廣泛的 FTP 操作支持,包括文件上傳、下載、目錄管理等。通過其流暢的 API 設計,你可以輕松地在 .NET 應用程序中集成 FTP 功能。
該文章在 2024/10/23 10:01:24 編輯過