本文將詳細(xì)介紹如何使用C#進(jìn)行SQLite表的基本操作,包括創(chuàng)建表、修改表結(jié)構(gòu)、刪除表和重命名表。這些操作是數(shù)據(jù)庫管理的基礎(chǔ),對(duì)于開發(fā)數(shù)據(jù)驅(qū)動(dòng)的應(yīng)用程序至關(guān)重要。
準(zhǔn)備工作
首先,確保你已經(jīng)安裝了 System.Data.SQLite
NuGet包。在你的C#文件頂部添加以下using語句:
using System;
using System.Data.SQLite;
連接到數(shù)據(jù)庫
在進(jìn)行任何表操作之前,我們需要先連接到數(shù)據(jù)庫。以下是一個(gè)建立連接的輔助方法:
public static SQLiteConnection ConnectToDatabase(string dbPath)
{
try
{
SQLiteConnection connection = new SQLiteConnection($"Data Source={dbPath};Version=3;");
connection.Open();
return connection;
}
catch (Exception ex)
{
Console.WriteLine($"連接數(shù)據(jù)庫時(shí)出錯(cuò):{ex.Message}");
return null;
}
}
創(chuàng)建表
創(chuàng)建表是最基本的操作之一。以下是創(chuàng)建表的方法:
public static void CreateTable(SQLiteConnection connection, string tableName, string[] columns)
{
try
{
string columnsDefinition = string.Join(", ", columns);
string sql = $"CREATE TABLE IF NOT EXISTS {tableName} ({columnsDefinition})";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
command.ExecuteNonQuery();
}
Console.WriteLine($"表 {tableName} 創(chuàng)建成功。");
}
catch (Exception ex)
{
Console.WriteLine($"創(chuàng)建表時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
string[] columns = {
"ID INTEGER PRIMARY KEY AUTOINCREMENT",
"Name TEXT NOT NULL",
"Age INTEGER"
};
CreateTable(connection, "Users", columns);
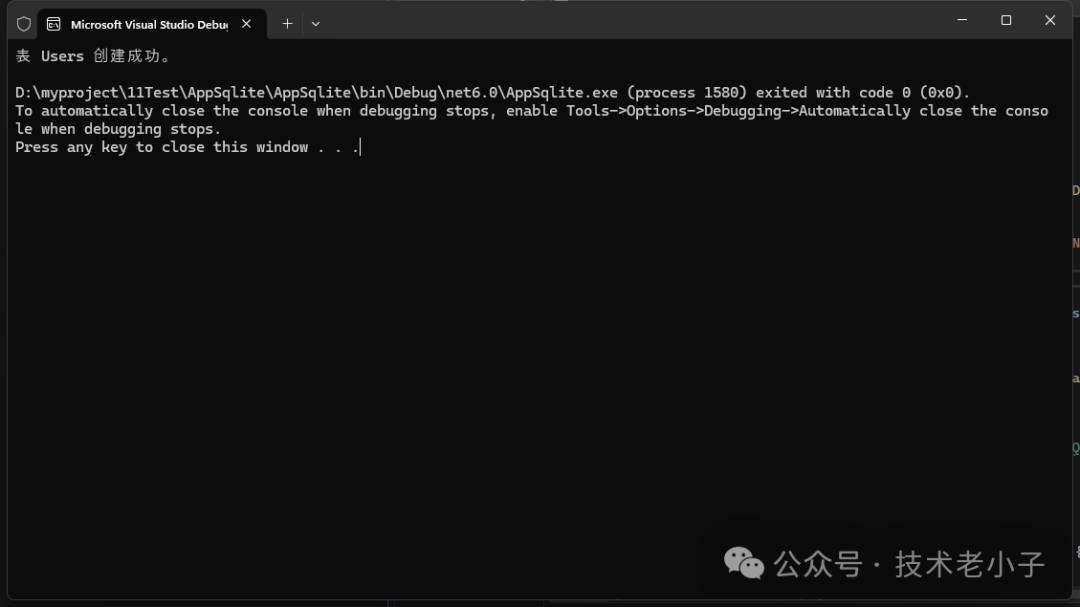
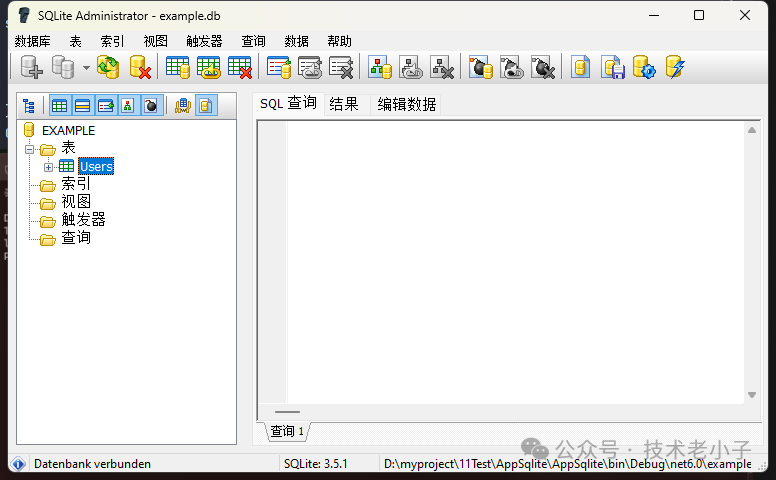
修改表結(jié)構(gòu)
修改表結(jié)構(gòu)包括添加列、刪除列等操作。SQLite對(duì)表結(jié)構(gòu)的修改有一些限制,主要支持添加列操作。
添加列
public static void AddColumn(SQLiteConnection connection, string tableName, string columnDefinition)
{
try
{
string sql = $"ALTER TABLE {tableName} ADD COLUMN {columnDefinition}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
command.ExecuteNonQuery();
}
Console.WriteLine($"列 {columnDefinition} 添加成功。");
}
catch (Exception ex)
{
Console.WriteLine($"添加列時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
AddColumn(connection, "Users", "Email TEXT");
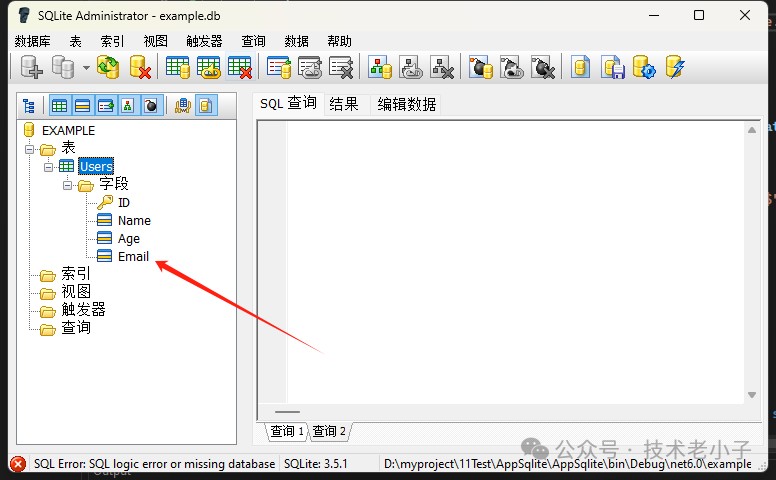
刪除表
刪除表的操作相對(duì)簡(jiǎn)單:
public static void DropTable(SQLiteConnection connection, string tableName)
{
try
{
string sql = $"DROP TABLE IF EXISTS {tableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
command.ExecuteNonQuery();
}
Console.WriteLine($"表 {tableName} 刪除成功。");
}
catch (Exception ex)
{
Console.WriteLine($"刪除表時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
DropTable(connection, "Users");
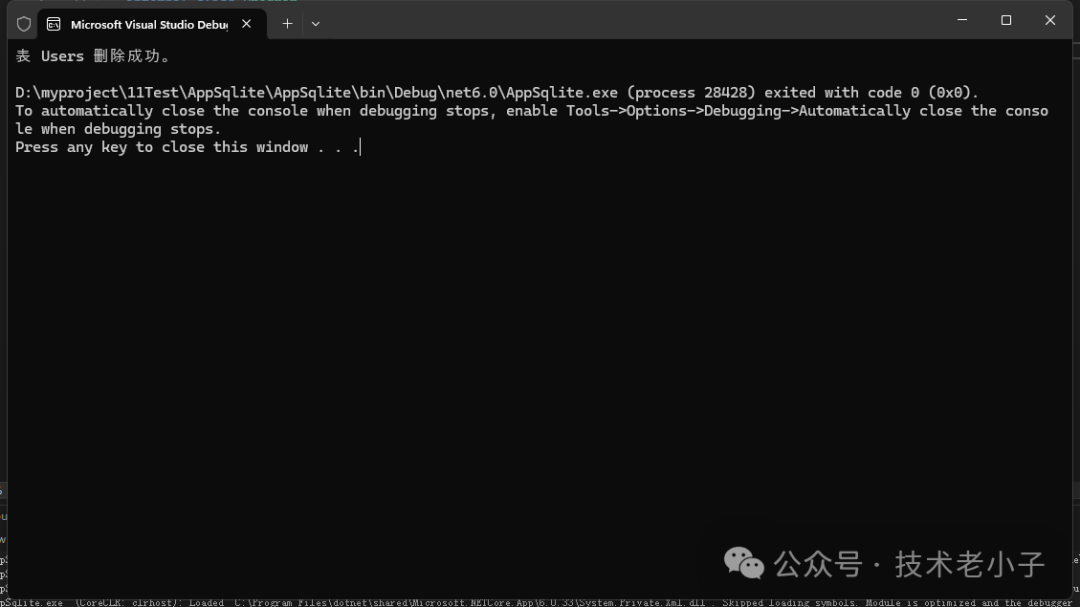
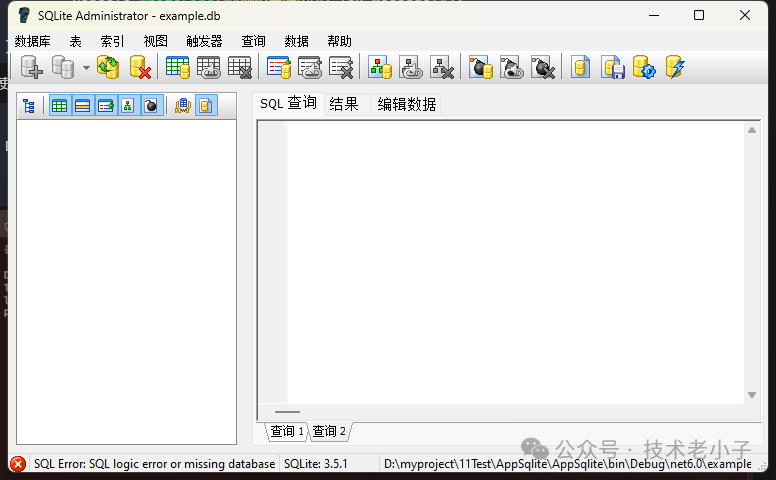
重命名表
SQLite支持使用 ALTER TABLE
語句來重命名表:
public static void RenameTable(SQLiteConnection connection, string oldTableName, string newTableName)
{
try
{
string sql = $"ALTER TABLE {oldTableName} RENAME TO {newTableName}";
using (SQLiteCommand command = new SQLiteCommand(sql, connection))
{
command.ExecuteNonQuery();
}
Console.WriteLine($"表 {oldTableName} 重命名為 {newTableName} 成功。");
}
catch (Exception ex)
{
Console.WriteLine($"重命名表時(shí)出錯(cuò):{ex.Message}");
}
}
使用示例:
RenameTable(connection, "Users", "Customers");
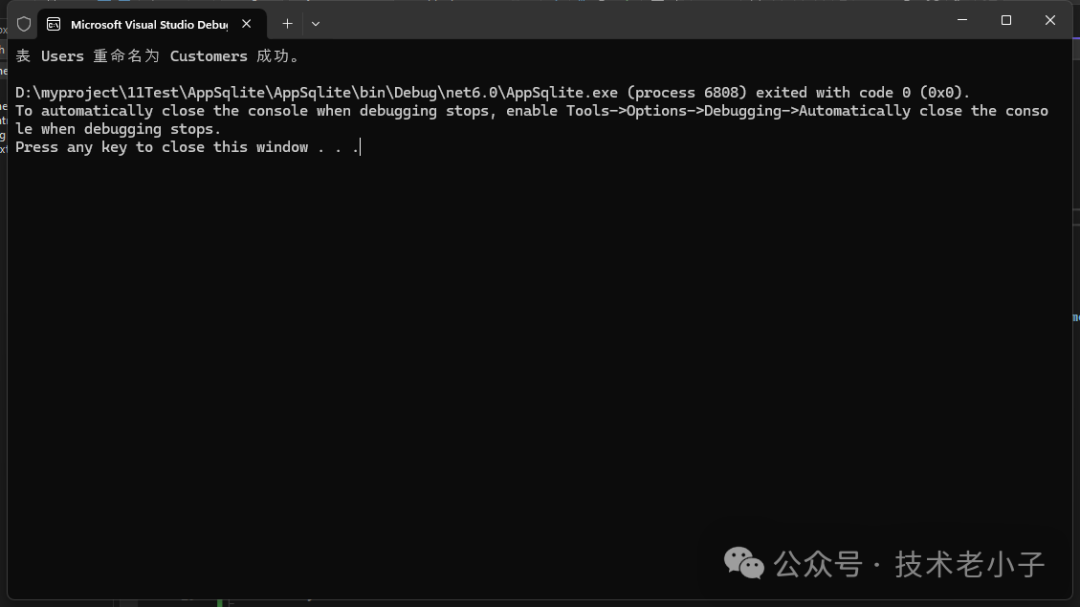
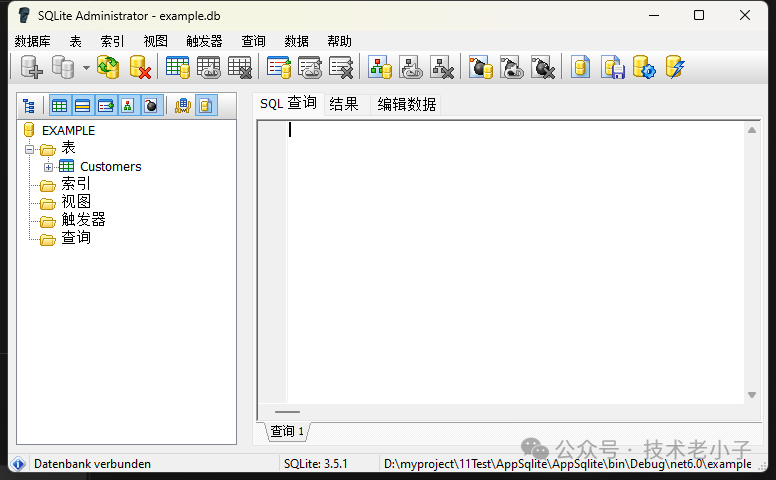
完整示例
以下是一個(gè)完整的示例,展示了如何使用上述所有方法:
using System;
using System.Data.SQLite;
class Program
{
static void Main(string[] args)
{
string dbPath = "C:\\example.db";
SQLiteConnection connection = ConnectToDatabase(dbPath);
if (connection != null)
{
// 創(chuàng)建表
string[] columns = {
"ID INTEGER PRIMARY KEY AUTOINCREMENT",
"Name TEXT NOT NULL",
"Age INTEGER"
};
CreateTable(connection, "Users", columns);
// 添加列
AddColumn(connection, "Users", "Email TEXT");
// 重命名表
RenameTable(connection, "Users", "Customers");
// 刪除表
DropTable(connection, "Customers");
// 關(guān)閉連接
connection.Close();
}
}
// 連接數(shù)據(jù)庫方法
public static SQLiteConnection ConnectToDatabase(string dbPath)
{
// 實(shí)現(xiàn)代碼...
}
// 創(chuàng)建表方法
public static void CreateTable(SQLiteConnection connection, string tableName, string[] columns)
{
// 實(shí)現(xiàn)代碼...
}
// 添加列方法
public static void AddColumn(SQLiteConnection connection, string tableName, string columnDefinition)
{
// 實(shí)現(xiàn)代碼...
}
// 刪除表方法
public static void DropTable(SQLiteConnection connection, string tableName)
{
// 實(shí)現(xiàn)代碼...
}
// 重命名表方法
public static void RenameTable(SQLiteConnection connection, string oldTableName, string newTableName)
{
// 實(shí)現(xiàn)代碼...
}
}
注意事項(xiàng)
SQLite對(duì)表結(jié)構(gòu)的修改有一些限制,例如不支持直接刪除或修改列。如果需要這些操作,通常的做法是創(chuàng)建一個(gè)新表,復(fù)制數(shù)據(jù),然后刪除舊表。
在實(shí)際應(yīng)用中,應(yīng)該考慮使用參數(shù)化查詢來防止SQL注入攻擊。
對(duì)于大型數(shù)據(jù)庫操作,考慮使用事務(wù)來確保數(shù)據(jù)完整性。
始終記得在完成操作后關(guān)閉數(shù)據(jù)庫連接。
結(jié)論
本文詳細(xì)介紹了使用C#進(jìn)行SQLite表操作的方法,包括創(chuàng)建表、修改表結(jié)構(gòu)、刪除表和重命名表。這些操作為進(jìn)一步的數(shù)據(jù)管理和操作奠定了基礎(chǔ)。在實(shí)際應(yīng)用中,你還需要考慮數(shù)據(jù)插入、查詢、更新和刪除等更多操作。
該文章在 2024/11/1 9:14:51 編輯過