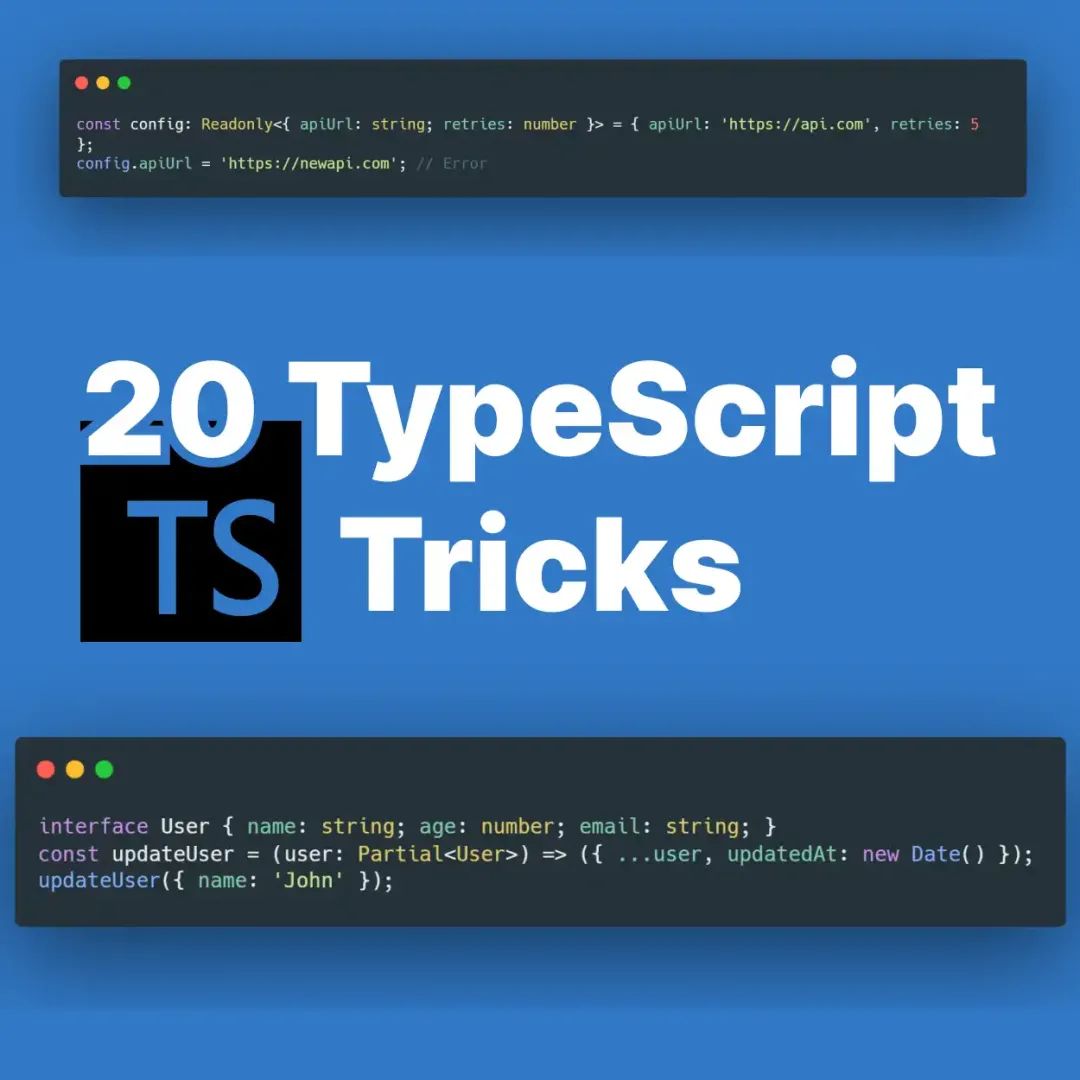
TypeScript 以類型安全及強大特性強化了 JavaScript,然而眾多開發(fā)者僅運用其基礎功能。在此為您呈現(xiàn)一份涵蓋 20 個高級 TypeScript 技巧的指南,這些技巧有助于提升生產(chǎn)力、代碼可維護性,利于提高代碼效率與可讀性。每個技巧均佐以實用代碼示例加以闡釋。
1. NonNullable:剔除 null 與 undefined 類型
type User = { name: string; age?: number | null };
const userAge: NonNullable<User['age']> = 30; // No null or undefined?
2. Partial:使所有屬性變?yōu)榭蛇x屬性
interface User {
name: string;
age: number;
email: string;
}
const updateUser = (user: Partial<User>) => ({ ...user, updatedAt: new Date() });
updateUser({ name: 'John' });
3. Readonly:強制實現(xiàn)只讀屬性
const config: Readonly<{ apiUrl: string; retries: number }> = {
apiUrl: 'https://api.com',
retries: 5,
};
config.apiUrl = 'https://newapi.com'; // Error
4. Mapped Types:動態(tài)地轉換現(xiàn)有類型
type Status = 'loading' | 'success' | 'error';
type ApiResponse<T> = { [K in Status]: T };
const response: ApiResponse<string> = { loading: 'Loading...', success: 'Done', error: 'Error' };
5. Optional Tuple Elements:使用變形 tuple 類型。
type UserTuple = [string, number?, boolean?];
const user1: UserTuple = ['Rob']; // Only name
6. Union Exhaustiveness:確保所有情況都被處理
type Status = 'open' | 'closed' | 'pending';
function handleStatus(status: Status) {
switch (status) {
case 'open':
return 'Opened';
case 'closed':
return 'Closed';
case 'pending':
return 'Pending';
default:
const exhaustiveCheck: never = status;
return exhaustiveCheck;
}
}
7. Omit:從類型中移除屬性
interface Todo {
title: string;
description: string;
completed: boolean;
}
type TodoPreview = Omit<Todo, 'description'>;
const todo: TodoPreview = { title: 'Learn TypeScript', completed: false };
8. Type Narrowing:使用 in 和 instanceof 來縮小類型
function processInput(input: string | number | { title: string }) {
if (typeof input === 'string') return input.toUpperCase();
if ('title' in input) return input.title.toUpperCase();
}
9. Conditional Types:應用條件邏輯
type IsString<T> = T extends string ? true : false;
type CheckString = IsString<'Hello'>; // true
10. 使用 as const 創(chuàng)建字面類型
const COLORS = ['red', 'green', 'blue'] as const;
type Color = (typeof COLORS)[number]; // 'red' | 'green' | 'blue'
11. Extract and Exclude:過濾聯(lián)合類型
type T = 'a' | 'b' | 'c';
type OnlyAOrB = Extract<T, 'a' | 'b'>; // 'a' | 'b'
12. 自定義類型守衛(wèi)
function isString(input: any): input is string {
return typeof input === 'string';
}
13. Record:動態(tài)對象類型
type Role = 'admin' | 'user' | 'guest';
const permissions: Record<Role, string[]> = { admin: ['write'], user: ['read'], guest: ['view'] };
14. Index Signatures:添加動態(tài)屬性
class DynamicObject {
[key: string]: any;
}
const obj = new DynamicObject();
obj.name = 'Rob';
15. Never Type:用于詳盡的檢查
function assertNever(value: never): never {
throw new Error(`Unexpected: ${value}`);
}
16. Optional Chaining:使用可選鏈
const user = { profile: { name: 'John' } };
const userName = user?.profile?.name; // 'John'
17. Null 合并操作符
const input: string | null = null;
const defaultValue = input ?? 'Default'; // 'Default'
18. ReturnType:推導函數(shù)返回類型。
function getUser() {
return { name: 'John', age: 30 };
}
type UserReturn = ReturnType<typeof getUser>;
19. Generics:可變函數(shù)類型。
function identity<T>(value: T): T {
return value;
}
identity<string>('Hello'); // 'Hello'
20. Intersection Types:組合多個類型。
type Admin = { privileges: string[] };
type User = { name: string };
type AdminUser = Admin & User;
總結
這些技巧中的每一個都突出了編寫更簡潔、更可靠的 TypeScript 代碼的方法。使用這些技巧,你可以充分利用 TypeScript 的完整類型系統(tǒng),實現(xiàn)更安全、更易于維護的開發(fā)。
該文章在 2024/11/14 11:38:35 編輯過