在C#中,我們可以使用Windows Win32 API來對系統(tǒng)注冊表進行操作。注冊表是Windows操作系統(tǒng)中用來存儲配置信息的重要數(shù)據(jù)庫,我們可以通過C#來讀取、寫入和刪除注冊表中的鍵和值。
下面是一些使用C#調用系統(tǒng)Windows Win32 API注冊表操作的示例:
讀取注冊表鍵值
RegOpenKeyEx
用于打開指定的注冊表鍵。它的參數(shù)說明如下:
hKey:指定要打開的注冊表鍵的句柄。可以是以下值之一:
lpSubKey:要打開的注冊表鍵的名稱。例如:"SOFTWARE\MyApp"。
ulOptions:保留參數(shù),通常設置為0。
samDesired:指定打開注冊表鍵的訪問權限和選項??梢允且韵轮抵换蛩鼈兊慕M合:
KEY_READ(只讀)
KEY_WRITE(寫入)
KEY_ALL_ACCESS(完全訪問權限)
phkResult:用于接收打開的注冊表鍵的句柄。
函數(shù)返回值為整型,表示操作的結果。
RegQueryValueEx
用于檢索指定注冊表鍵的值。它的參數(shù)說明如下:
hKey:指定要查詢的注冊表鍵的句柄。
lpValueName:要查詢的注冊表值的名稱。
lpReserved:保留參數(shù),通常設置為0。
lpType:用于接收鍵值數(shù)據(jù)的類型。例如,REG_SZ、REG_DWORD等。
lpData:用于接收鍵值數(shù)據(jù)的緩沖區(qū)的指針。
lpcbData:指定lpData緩沖區(qū)的大小,以字節(jié)為單位。在調用函數(shù)之前,它指定lpData緩沖區(qū)的大小。在函數(shù)返回時,它包含實際寫入lpData緩沖區(qū)的字節(jié)數(shù)。
函數(shù)返回值為整型,表示操作的結果。
using System;
using Microsoft.Win32.SafeHandles;
using System.Runtime.InteropServices;
class Program
{
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegOpenKeyEx(
IntPtr hKey,
string lpSubKey,
int ulOptions,
int samDesired,
out IntPtr phkResult
);
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegQueryValueEx(
IntPtr hKey,
string lpValueName,
int lpReserved,
out uint lpType,
IntPtr lpData,
ref uint lpcbData
);
static void Main()
{
IntPtr hKey;
IntPtr phkResult;
uint lpType;
uint lpcbData = 1024;
IntPtr lpData = Marshal.AllocHGlobal((int)lpcbData);
// 打開注冊表鍵
int result = RegOpenKeyEx(
new IntPtr((int)RegistryHive.CurrentUser),
"SOFTWARE\\MyApp",
0,
0x20019, // KEY_READ
out phkResult
);
// 讀取鍵值
result = RegQueryValueEx(
phkResult,
"MySetting",
0,
out lpType,
lpData,
ref lpcbData
);
string value = Marshal.PtrToStringUni(lpData);
// 輸出鍵值
Console.WriteLine(value);
// 釋放內存
Marshal.FreeHGlobal(lpData);
// 關閉注冊表鍵
SafeRegistryHandle safeHandle = new SafeRegistryHandle(phkResult, true);
safeHandle.Dispose();
}
}
讀取之前需要先創(chuàng)建一個Key
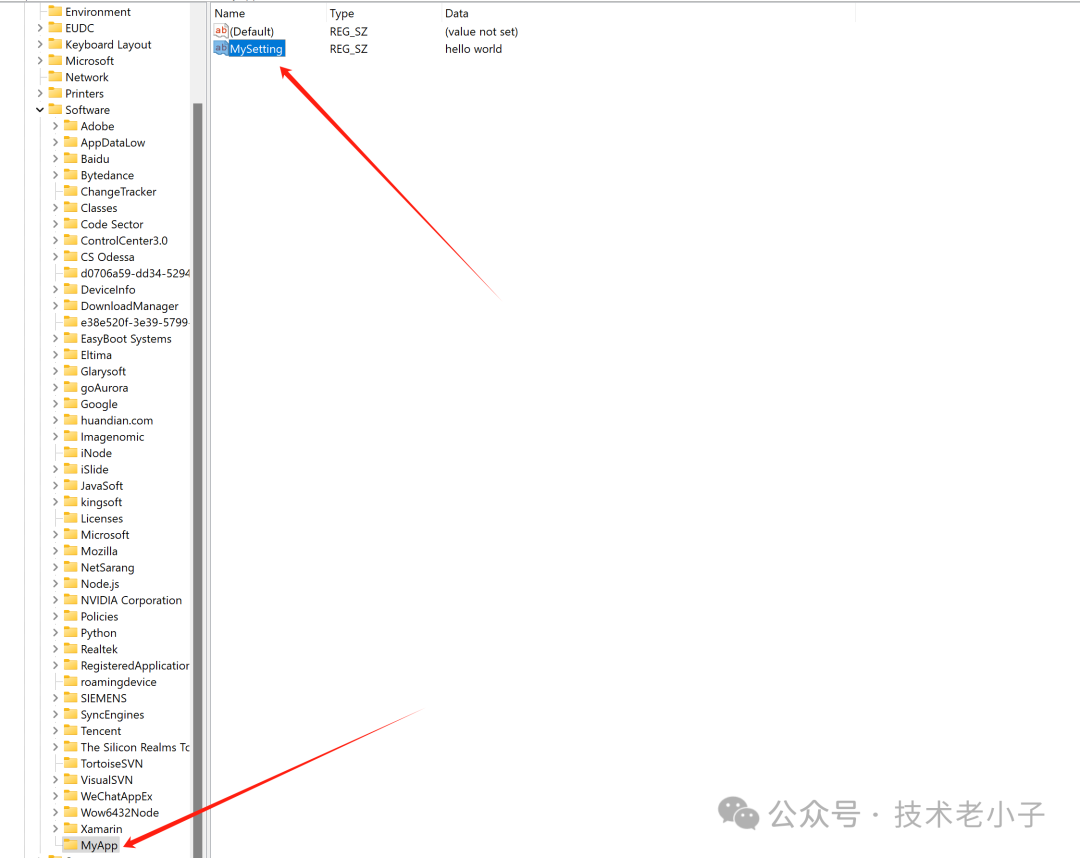
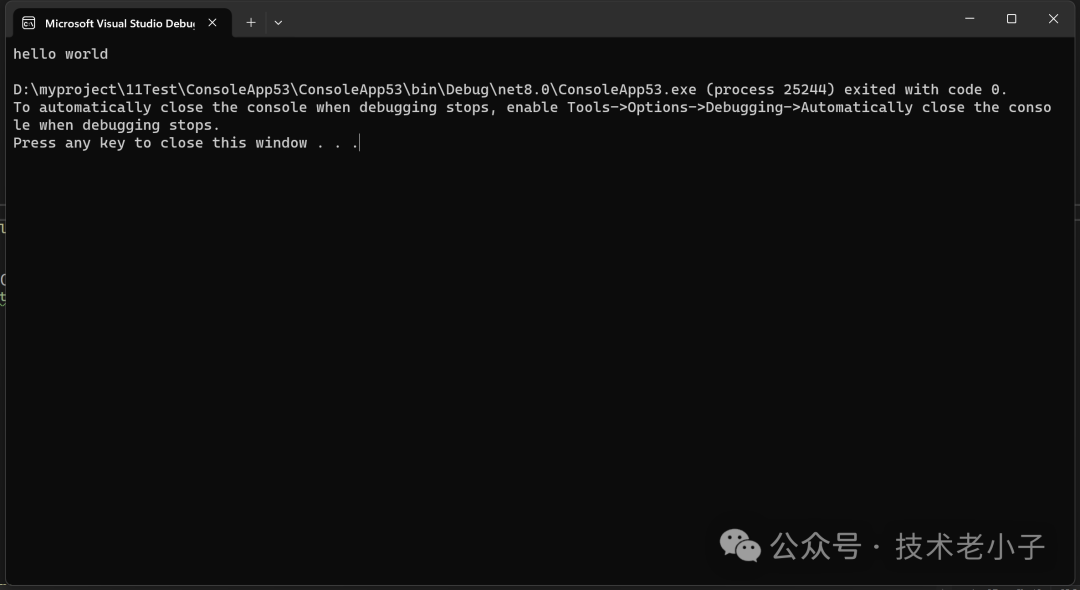
寫入注冊表鍵值
RegSetValueEx
用于設置指定注冊表鍵的值。它的參數(shù)說明如下:
hKey:指定要設置值的注冊表鍵的句柄。
lpValueName:要設置的注冊表值的名稱。
reserved:保留參數(shù),通常設置為0。
dwType:指定要設置的值的數(shù)據(jù)類型。可以是以下值之一:
lpData:指向包含要設置的值的數(shù)據(jù)的緩沖區(qū)的指針。
cbData:指定lpData緩沖區(qū)的大小,以字節(jié)為單位。
函數(shù)返回值為整型,表示操作的結果。
using System;
using Microsoft.Win32.SafeHandles;
using System.Runtime.InteropServices;
class Program
{
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegCreateKeyEx(
IntPtr hKey,
string lpSubKey,
int reserved,
string lpClass,
int dwOptions,
int samDesired,
IntPtr lpSecurityAttributes,
out IntPtr phkResult,
out int lpdwDisposition
);
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegSetValueEx(
IntPtr hKey,
string lpValueName,
int reserved,
int dwType,
string lpData,
int cbData
);
static void Main()
{
IntPtr hKey;
IntPtr phkResult;
int lpdwDisposition;
// 創(chuàng)建或打開注冊表鍵
int result = RegCreateKeyEx(
new IntPtr((int)RegistryHive.CurrentUser),
"SOFTWARE\\MyApp",
0,
null,
0,
0x20006, // KEY_WRITE
IntPtr.Zero,
out phkResult,
out lpdwDisposition
);
// 寫入鍵值
result = RegSetValueEx(
phkResult,
"MySetting",
0,
1, // REG_SZ
"123",
6
);
// 關閉注冊表鍵
SafeRegistryHandle safeHandle = new SafeRegistryHandle(phkResult, true);
safeHandle.Dispose();
}
}
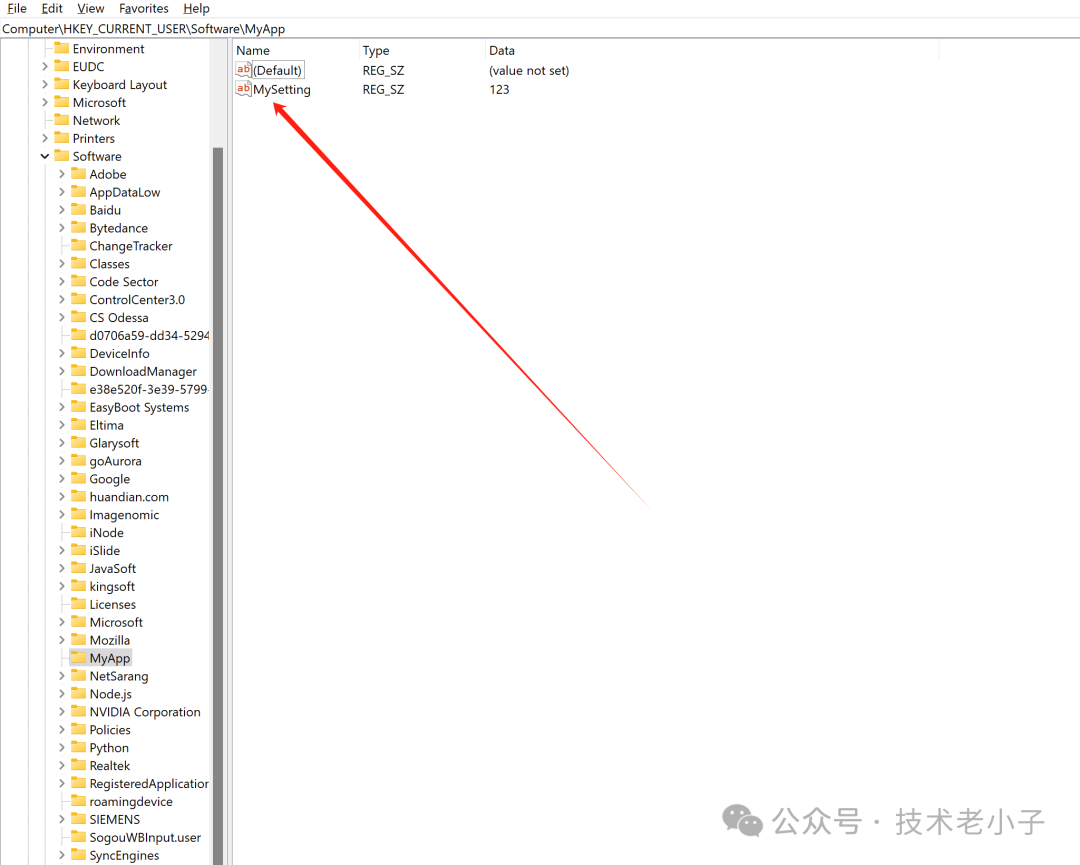
刪除注冊表鍵值
class Program
{
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegOpenKeyEx(
IntPtr hKey,
string lpSubKey,
int ulOptions,
int samDesired,
out IntPtr phkResult
);
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegDeleteValue(
IntPtr hKey,
string lpValueName
);
static void Main()
{
IntPtr hKey;
IntPtr phkResult;
// 打開注冊表鍵
int result = RegOpenKeyEx(
new IntPtr((int)RegistryHive.CurrentUser),
"SOFTWARE\\MyApp",
0,
0x20006, // KEY_WRITE
out phkResult
);
// 刪除鍵值
result = RegDeleteValue(
phkResult,
"MySetting"
);
// 關閉注冊表鍵
SafeRegistryHandle safeHandle = new SafeRegistryHandle(phkResult, true);
safeHandle.Dispose();
}
}
刪除注冊表鍵
class Program
{
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegOpenKeyEx(
IntPtr hKey,
string lpSubKey,
int ulOptions,
int samDesired,
out IntPtr phkResult
);
// 定義Win32 API函數(shù)
[DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int RegDeleteKey(
IntPtr hKey,
string lpSubKey
);
static void Main()
{
IntPtr hKey;
IntPtr phkResult;
// 打開注冊表鍵
int result = RegOpenKeyEx(
new IntPtr((int)RegistryHive.CurrentUser),
"SOFTWARE",
0,
0x20019, // KEY_READ
out phkResult
);
// 刪除注冊表鍵
result = RegDeleteKey(
phkResult,
"MyApp"
);
// 關閉注冊表鍵
SafeRegistryHandle safeHandle = new SafeRegistryHandle(phkResult, true);
safeHandle.Dispose();
}
}
通過以上示例,我們可以看到如何使用C#調用系統(tǒng)Windows Win32 API注冊表操作。需要注意的是,對注冊表的操作需要謹慎,不當?shù)牟僮骺赡軙е孪到y(tǒng)故障,建議在操作注冊表時謹慎處理。
該文章在 2024/11/15 11:40:21 編輯過