前言
HTML 是一種標記語言,而每個 Web 網(wǎng)頁都是一個 HTML 文件。當需要將HTML文件轉(zhuǎn)為PDF或圖片文件時,可以通過什么方法實現(xiàn)呢?又如何通過編程方式將HTML為PDF或圖片文件。本文將介紹 wkhtmltopdf 在 .NET 中的 C# 實現(xiàn)。
wkhtmltopdf
1、概述
wkhtmltopdf 是一個開源免費命令行工具,它使用 Webkit 將 HTML 轉(zhuǎn)換為 PDF 和圖像。由于其是使用命令行交互,所以需要通過編寫調(diào)用外部命令的方實現(xiàn)。
2、附錄
//Github 地址
https://github.com/wkhtmltopdf/wkhtmltopdf
// 官方網(wǎng)站下載 根據(jù)系統(tǒng)下載對應(yīng)版本
https://wkhtmltopdf.org/downloads.html
3、文件
// 需將下面的文件放我們程序目錄下
wkhtmltoimage.exe -- 轉(zhuǎn)圖片
wkhtmltopdf.exe --轉(zhuǎn)PDF
wkhtmltox.dll
實現(xiàn)
1、定義轉(zhuǎn)換接口
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
public interface IConverterEngine
{
/// <summary>
/// wkhtmltopdf 工具路徑
/// </summary>
string ConverterPath { get; }
/// <summary>
/// 轉(zhuǎn)換類型
/// </summary>
int EngineType { get; }
/// <summary>
/// 轉(zhuǎn)換
/// </summary>
/// <param name="htmlPath">HTML文件路徑</param>
/// <param name="outputPath">輸出文件路徑</param>
/// <returns></returns>
bool Convert(string htmlPath, string outputPath);
}
}
2、實現(xiàn) PDF 轉(zhuǎn)換
using System.Diagnostics;
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
public class ConverterPDF:IConverterEngine
{
/// <summary>
/// wkhtmltopdf 工具路徑
/// </summary>
public string ConverterPath { get; }
/// <summary>
/// 轉(zhuǎn)換類型
/// </summary>
public int EngineType { get; } = 1;
/// <summary>
///
/// </summary>
/// <param name="converterPath"></param>
public ConverterPDF(string converterPath)
{
ConverterPath = converterPath;
}
/// <summary>
///
/// </summary>
/// <param name="htmlPath"></param>
/// <param name="outputPath"></param>
/// <returns></returns>
public bool Convert(string htmlPath, string outputPath)
{
try
{
var ticks = DateTime.UtcNow.Ticks;
string optionSwitches = "";
#region 頁眉
// 在頁眉的居中部分顯示頁眉文本
optionSwitches += "--header-center 輸出文件 ";
// 在頁眉下方顯示一條直線分隔正文
optionSwitches += "--header-line ";
// 頁眉與正文之間的距離(默認為零)
optionSwitches += "--header-spacing 1 ";
#endregion
#region 頁面
// 使用的打印介質(zhì)類型,而不是屏幕
optionSwitches += "--print-media-type ";
// 邊距
optionSwitches += "--margin-top 40mm --margin-bottom 10mm --margin-right 10mm --margin-left 10mm ";
// 紙張大小
optionSwitches += "--page-size A4 ";
#endregion
#region 頁腳
// 在頁腳上方顯示一條直線分隔正文
optionSwitches += "--footer-line ";
// 在頁腳的居中部分顯示頁腳文本
optionSwitches += "--footer-center \"[page] of [topage]\" ";
#endregion
Process process = new Process();
process.StartInfo.UseShellExecute = true;
process.StartInfo.FileName = this.ConverterPath;
process.StartInfo.Arguments = $"{optionSwitches} \"{htmlPath}\" \"{outputPath}\" ";
process.Start();
}
catch (Exception ex)
{
throw new Exception("轉(zhuǎn)PDF出錯", ex);
}
return true;
}
}
}
3、實現(xiàn)圖片轉(zhuǎn)換
using System.Diagnostics;
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
public class ConverterImage:IConverterEngine
{
/// <summary>
/// wkhtmltopdf 工具路徑
/// </summary>
public string ConverterPath { get; }
/// <summary>
/// 轉(zhuǎn)換類型
/// </summary>
public int EngineType { get; } = 2;
/// <summary>
///
/// </summary>
/// <param name="converterPath"></param>
public ConverterImage(string converterPath)
{
ConverterPath = converterPath;
}
/// <summary>
///
/// </summary>
/// <param name="htmlPath"></param>
/// <param name="outputPath"></param>
/// <returns></returns>
public bool Convert(string htmlPath, string outputPath)
{
try
{
Process process = new Process();
process.StartInfo.UseShellExecute = true;
process.StartInfo.FileName = this.ConverterPath;
process.StartInfo.Arguments = $"\"{htmlPath}\" \"{outputPath}\" ";
process.Start();
}
catch (Exception ex)
{
throw new Exception("轉(zhuǎn)換圖片出錯", ex);
}
return true;
}
}
}
4、轉(zhuǎn)換調(diào)用
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
internal class Program
{
static void Main(string[] args)
{
var ticks = DateTime.UtcNow.Ticks;
string outputpdf = $"{AppDomain.CurrentDomain.BaseDirectory}{ticks}.pdf";
string htmlPath = $"{AppDomain.CurrentDomain.BaseDirectory}test.html";
string convertPath= $"{AppDomain.CurrentDomain.BaseDirectory}wkhtmltopdf.exe";
ConverterPDF converter = new ConverterPDF(convertPath);
converter?.Convert(htmlPath, outputpdf);
Thread.Sleep(1000);
string outputpng = $"{AppDomain.CurrentDomain.BaseDirectory}{ticks}.png";
string convertImagePath = $"{AppDomain.CurrentDomain.BaseDirectory}wkhtmltoimage.exe";
ConverterImage converterImage = new ConverterImage(convertImagePath);
converterImage.Convert(htmlPath, outputpng);
Console.ReadKey();
}
}
}
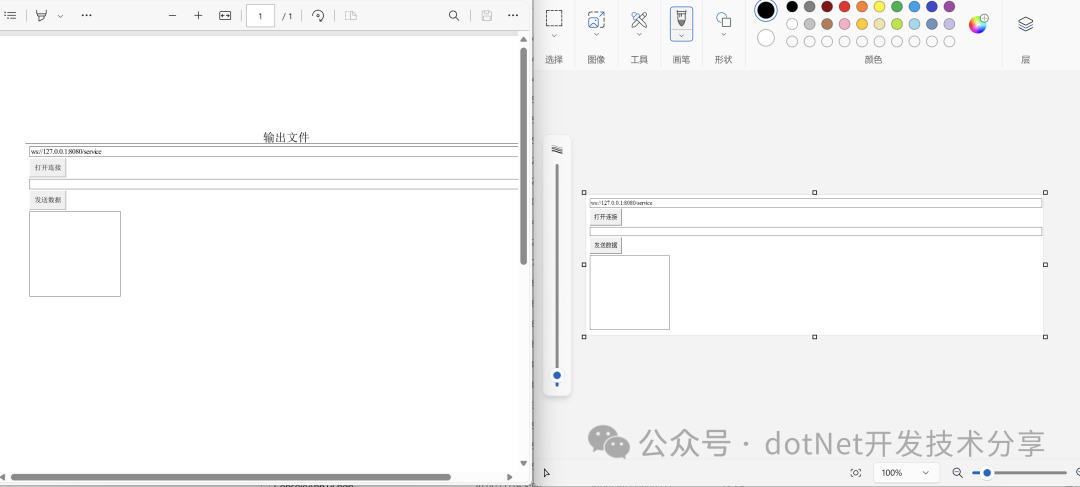
5、HTML文件
<!DOCTYPE HTML>
<html>
<head>
<meta charset="gbk">
<title>測式文件</title>
</head>
<body>
<div id="sse">
<input id="url" size=200 value="ws://127.0.0.1:8080/service" /><button id="btn1" onclick="changewebsocket(this)" tt=1>打開連接</button><br>
<input id="msg" size=200 value='測試內(nèi)容'/>
<button onclick="sendmsg()">發(fā)送數(shù)據(jù)</button><br>
<textarea id="onmsg" rows="10" cols="30"></textarea>
</div>
</body>
</html>
小結(jié)
本文通過實現(xiàn)示例,描述了 wkhtmltopdf 在 C# 中的實現(xiàn)方式。通過示例,根據(jù)您的需求,修改對應(yīng)代碼使其適應(yīng)各種文檔格式。
該文章在 2024/11/18 9:05:40 編輯過